Transitioning from SQL Server to PostgreSQL in an ASP.NET Core Application.
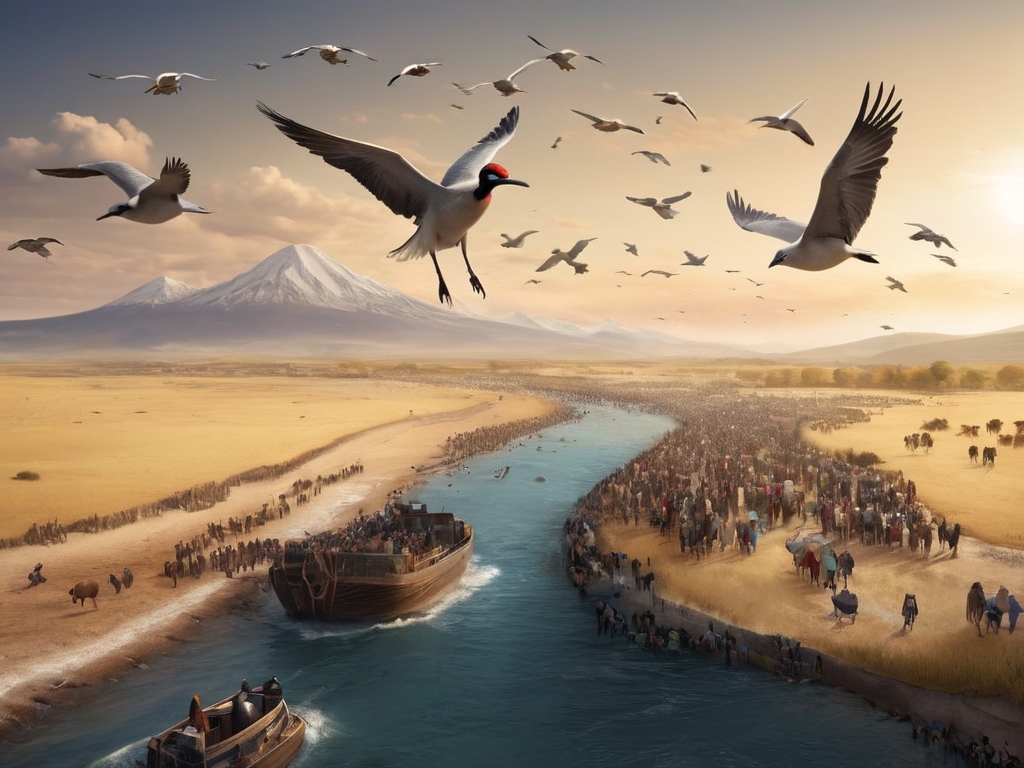
Note to the Reader
This article is for developers with an existing ASP.NET Core Entity Framework based application currently utilizing SQL Server database for user authentication. If you’re considering migrating to PostgreSQL, this guide is designed to provide you with a clear and effective roadmap for a seamless transition."
Overview
In the ever-evolving landscape of database management, the decision to migrate from SQL Server to PostgreSQL marks a strategic move towards enhanced flexibility, cost efficiency, and scalability. This comprehensive guide aims to demystify the migration process, providing ASP.NET Core developers with a roadmap to smoothly transition their applications to the PostgreSQL ecosystem
Tech
-
ASP.NET Core MVC: A powerful and open-source web framework for building modern, dynamic, and cross-platform web applications using the ASP.NET framework.
-
Identity (ASP.NET Core Identity): A membership system that adds login functionality to ASP.NET Core applications, providing user authentication, authorization, and account management features.
-
Entity Framework: A powerful and versatile Object-Relational Mapping (ORM) framework in .NET, simplifying database interactions by allowing developers to work with database entities using familiar object-oriented principles."
-
PostgreSQL: An advanced, open-source relational database management system known for its extensibility, standards compliance, and robust support for complex queries, making it a popular choice for web applications.
Need for Migration
Migrating from SQL Server to PostgreSQL in an application may be driven by various reasons, including:
-
Cost Efficiency: PostgreSQL is open-source, eliminating licensing costs associated with SQL Server, making it an economical choice for budget-conscious projects.
-
Platform Agnosticism: PostgreSQL is cross-platform, providing flexibility in choosing operating systems, whereas SQL Server is predominantly associated with Windows environments.
-
Community Support: PostgreSQL has a robust and active open-source community, offering extensive support, frequent updates, and a wealth of resources for developers.
-
Global Presence: PostgreSQL is widely adopted globally, leading to a diverse user community and a broad range of use cases, demonstrating its suitability for different scenarios.
-
Vendor Independence: Choosing PostgreSQL reduces dependency on a single vendor, fostering independence and allowing for greater control over the database environment.
Considering these factors, businesses and developers may opt to migrate from SQL Server to PostgreSQL to capitalize on the advantages offered by PostgreSQL’s open-source nature, community support, and versatile features.
Step 1: Prepare for Migration
Your existing SQL Server Entity framework based project might have migration codes. This needs to be deleted. This ensures that the new PostgreSQL migrations will be generated from scratch.
# Delete the Migration folder
rm -rf Migrations
Step 2: Add Necessary NuGet Packages
To enable PostgreSQL support, add the required NuGet packages to your project file:
<!-- Microsoft.VisualStudio.Web.CodeGeneration.Design -->
<PackageReference Include="Microsoft.VisualStudio.Web.CodeGeneration.Design" Version="7.0.11" />
<!-- Npgsql.EntityFrameworkCore.PostgreSQL -->
<PackageReference Include="Npgsql.EntityFrameworkCore.PostgreSQL" Version="7.0.0" />
Use the dotnet restore
command to fetch the newly added packages.
dotnet restore
Step 3: Update Connection String
Adjust the connection string in your appsettings.json
or appsettings.Development.json
to reflect the PostgreSQL server details:
"DefaultConnection": "Host=localhost;Port=5433;Database=authentication_app;Username=postgres;Password=<YOURPASSWORD>;",
Ensure that the host, port, database name, username, and password match your PostgreSQL setup.
Step 4: Modify DbContext Configuration
Replace the SQL Server configuration in your Startup.cs
file with PostgreSQL configuration. Locate the code that configures the DbContext, and replace it as follows:
// Replace the existing SQL Server configuration
builder.Services.AddDbContext<AuthenticationAppDbContext>(options => options.UseSqlServer(connectionString));
// With the new PostgreSQL configuration
builder.Services.AddDbContext<IdentityContext>(options => options.UseNpgsql(connectionString));
This change ensures that your application now uses PostgreSQL as the underlying database.
Conclusion
Congratulations! You’ve successfully migrated your ASP.NET Core MVC application from SQL Server to PostgreSQL. Ensure to thoroughly test your application to verify that the migration was successful, and your functionalities remain intact.
By following these steps, you’ve embraced the power of PostgreSQL, providing your application with a robust and scalable database solution.
Post Migration View
Source Code
Download or clone the project code from https://github.com/deepakkumpala/User-Authentication-App
Happy coding!