Enhancing IoT Security and Reliability - Importance of loading user profiles
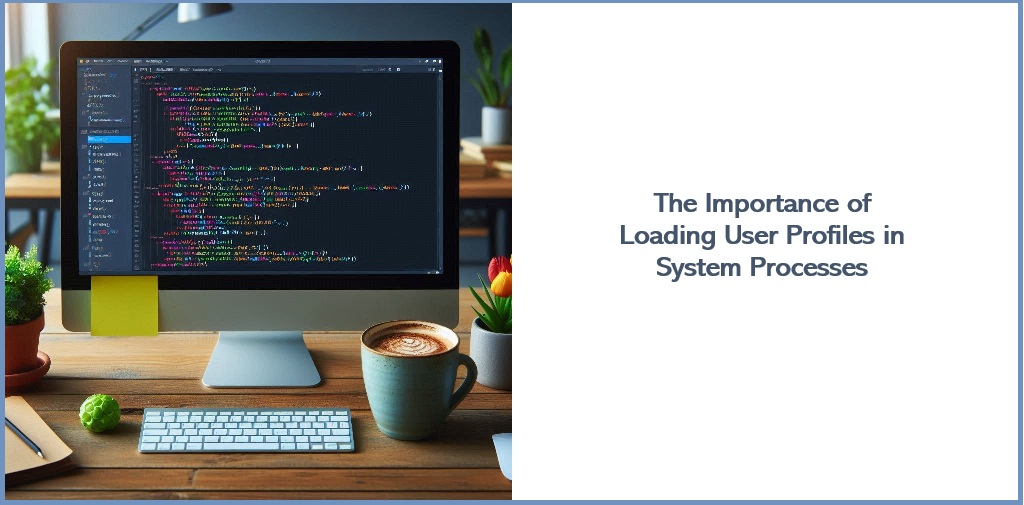
Overview
When developing IoT solutions for customers, managing device security and user profiles is critical, especially when running processes with elevated privileges or performing system-level tasks.
Suppose you are developing a small application that needs to make configuration changes on IoT devices. In such cases, you might write code to run system-level commands or scripts to manage tasks like network settings, certificate installations, or device-specific configurations. However, simply executing these commands without considering the user context or security requirements can lead to unpredictable results.
For example, you might start by writing a basic console code to launch a command, similar to the following:
ProcessStartInfo processStartInfo = new ProcessStartInfo
{
FileName = @"C:\Windows\System32\cmd.exe",
RedirectStandardOutput = true,
RedirectStandardError = true,
UseShellExecute = false
};
At first glance, this code may seem sufficient for executing tasks. But when dealing with IoT devices, certain key elements, such as user profile management and elevated permissions, are often overlooked. If your application needs access to user-specific settings, certificates, or requires admin privileges to modify system configurations, this approach may fail.
To address these challenges, a more robust code structure is necessary, like the one shown below:
ProcessStartInfo psi = new ProcessStartInfo
{
FileName = @"C:\Windows\System32\cmd.exe",
Arguments = $"/k \"{filePath}\"",
UseShellExecute = false,
CreateNoWindow = false,
Verb = "runas",
Domain = "",
LoadUserProfile = true,
UserName = "Admin"
};
By loading the user profile and ensuring the process runs with the appropriate privileges, you can better manage IoT device configurations, ensuring security and reliability.
LoadUserProfile
In the code snippet, LoadUserProfile = true
is explicitly set, ensuring that the user’s profile is loaded when the process is executed. This feature is crucial for IoT devices where:
- User-specific settings and configurations (such as network settings, certificates, or user-specific files) are required.
- Device-specific IoT solutions often need to perform tasks tied to a specific user’s environment, such as accessing user credentials, reading certificates, or applying user-defined settings.
Without loading the user profile (as is the case in the initial code), the process may run in a restricted environment, limiting access to these resources. As a result, critical operations that depend on the user profile may fail, leading to unpredictable behavior or system errors.
For example, an IoT device that authenticates against a user’s certificate might fail to access that certificate without loading the profile, disrupting secure communications.
Benefit of Arguments = $"/k"
The use of Arguments = $"/k "{filePath}"" in this code ensures that the command window remains open after the specified command is executed. This can be very beneficial during the development and troubleshooting phases of IoT solutions, as it allows you to:
View the output or errors of the executed command without the window closing immediately, which helps in diagnosing issues or understanding the behavior of the process. Monitor real-time execution of configuration scripts, providing better visibility and control over the process, especially when handling system-level tasks like network configuration or certificate installation.
Elevated Privileges with Verb = "runas"
The code also uses Verb = "runas"
, which is key for running the process with administrator privileges. In IoT device management, many operations such as updating system settings, installing certificates, or configuring low-level device behavior require elevated permissions.
The code does not explicitly include this functionality. It may cause issues when running commands that need higher access levels, leading to failures in critical tasks like security provisioning or system updates.
Explicit Control over User Context
The code also provides control over which user account runs the process through UserName = "Admin"
. In the context of IoT solutions, you often need to ensure that certain commands are run under specific user accounts with the appropriate permissions. This control ensures:
- The correct user profile is loaded.
- The necessary privileges are applied, which helps in managing sensitive device actions like installing user certificates or running setup scripts.
The initial code does not specify any user information, which might default to the current logged-in user or a system account. This could result in unpredictable behavior or security issues.
Visibility and Diagnostics
The improved code hides the window (CreateNoWindow = true
). For debugging and diagnostics during IoT development, having visibility into what the process is doing can be beneficial. This is particularly important in the early stages of IoT development, where understanding failures or issues in real-time is necessary.
Benefits for Saving the User Profile on Devices
In IoT solutions, user profiles often contain critical data such as:
- Network configurations, certificates for secure communication, and encryption keys.
- Device settings linked to the user that can ensure proper operation when multiple users share a device or when specific users require customized settings.
By using the code, which ensures the user profile is loaded, IoT developers can:
- Safeguard user-specific settings.
- Avoid operational failures due to missing user contexts.
- Ensure persistent and secure connections between IoT devices and back-end services (like cloud platforms), especially if user authentication or certificate-based security is involved.
For instance, if an IoT device relies on user certificates stored within the user profile to authenticate with an IoT hub, failing to load that profile (as in the initial code) would prevent the device from connecting securely, leading to potential outages or vulnerabilities.
Conclusion
In summary, the improved code is better suited for IoT solutions where user profiles need to be loaded to access user-specific resources, handle certificates, and manage security-sensitive tasks. The original code, while more lightweight, lacks key features like loading user profiles and managing privileges, which are essential for secure and reliable IoT device operations. When developing IoT solutions, these factors are critical to ensure devices function as expected in multi-user environments or when handling sensitive tasks like security provisioning.